Today I am going to teach you how to make a simple Audio Player in HTML 5, JavaScript, and CSS.
As streaming is increasingly being adopted by users, online media players have become essential for consuming media on the internet. Music/ Audio players allow one to enjoy music in any browser and supports a lot of the features of an offline music player.
We will be creating a audio player with a clean user interface that can be used to play music in the browser. We will also implement features like seeking and volume control.
We will start by creating the HTML layout first that defines the structure of the player, make it look good by styling using CSS and then write the player logic for all the functions in JavaScript.
To follow this tutorial (audio player in HTML, JavaScript, CSS), be sure to download notepad++ being as it is mostly used in HTML, JavaScript, and CSS.
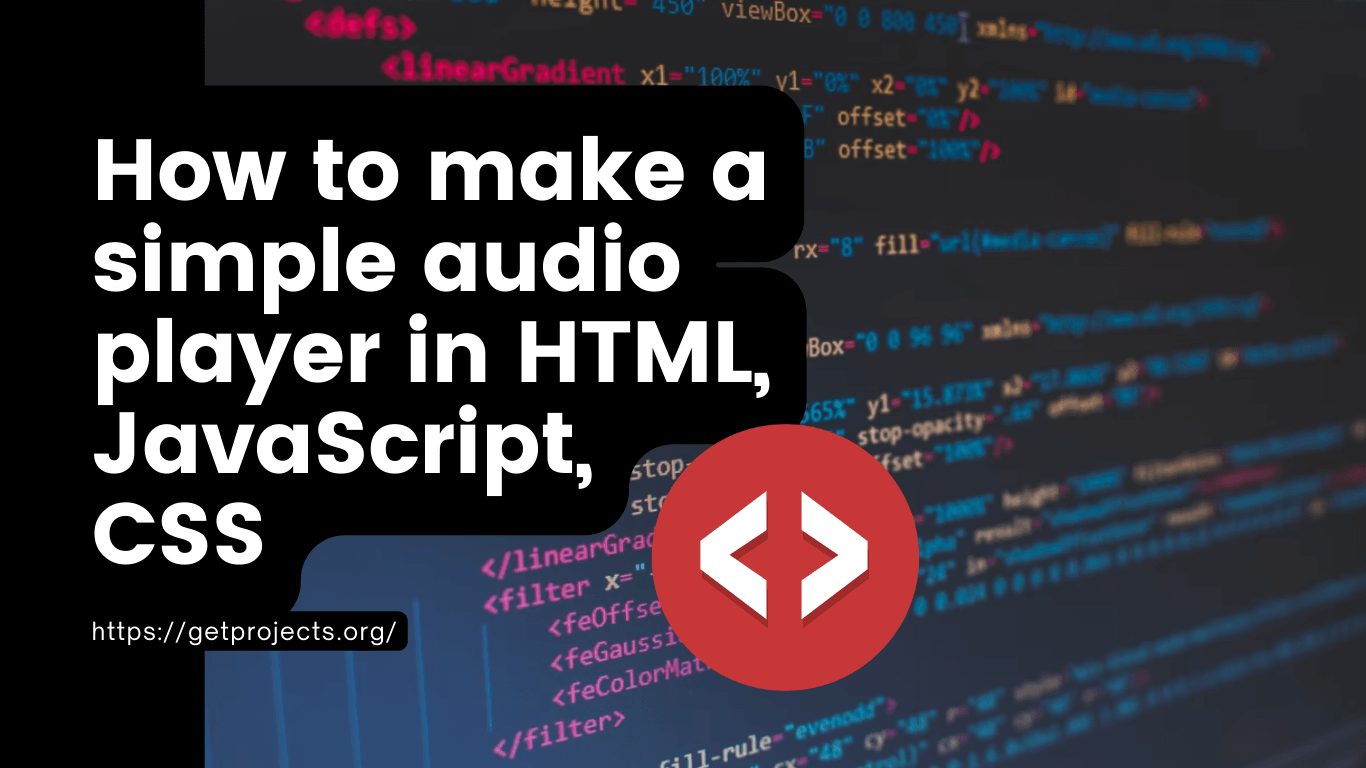
Table of Contents
Creating an audio player in HTML, JavaScript, and CSS is a common task for web developers. You can achieve this by using the HTML <audio>
element for the audio source, JavaScript for controlling playback, and CSS for styling. Here’s a step-by-step guide to creating a basic audio player:
Let’s begin
First create 3 important files, such as:
- HTML (player.html),
- JavaScript (player.js),
- CSS (player.css).
Also, we need to create two folders such as:
- Audio (Save your Audio file here, A file you want to play)
- Textures (To keep files (images) like, background of the player, and some button images like play, stop, pause, replay, volume icon of the player).
Read Also: How to Minify CSS, HTML, and JavaScript Files in WordPress
Code for simple audio player in HTML, JavaScript, CSS
Code for HTML File (player.html)
<html> <head> <script type="text/javascript" src="player.js"></script> <link rel="stylesheet" type="text/css" href="player.css"> </head> <body> <div id="wrapper"> <div id='player'> <audio id="music_player"> <source src="Audio/Your_Audio.mp3"/> </audio> <input type="image" src="Textures/play.png" onclick="play_aud()" id="play_button"> <input type="image" src="Textures/pause.png" onclick="pause_aud()" id="play_button"> <input type="image" src="Textures/stop.png" onclick="stop_aud()" id="play_button"> <input type="image" src="Textures/replay.png" onclick="replay_aud()" id="play_button"> <img src="Textures/volume.png" id="vol_img"> <input type="range" id="change_vol" onchange="change_vol()" step="0.05" min="0" max="1" value="1"> </div> </div> </body> </html>
Code for CSS file (player.css)
body { width:100%; margin:0 auto; padding:0px; font-family:helvetica; background-color:#00000F; } #wrapper { text-align:center; margin:0 auto; padding:50px; width:995px; } #player { background-color:#4CBB17; width:400px; margin-left:300px; padding:5px; box-sizing:border-box; background:url(Textures/background.png); } input[type="image"] { float:left; height:20px; margin-left:2px; margin-right:5px; margin-top:2px; } #vol_img { margin-left:150px; width:20px; }
Code for the JavaScript file (player.js)
document.addEventListener("DOMContentLoaded", function() { startplayer(); }, false); var player; function startplayer() { player = document.getElementById('music_player'); player.controls = false; } function play_aud() { player.play(); } function pause_aud() { player.pause(); } function stop_aud() { player.pause(); player.currentTime = 0; } function replay_aud() { player.loop = true; } function change_vol() { player.volume=document.getElementById("change_vol").value; }
Your Simple Audio Player in html is ready to play. To run your project, open player.html file
Follow the link below to Download the Project, containing all the tutorial files.
Round 2: Advance Version of audio player in HTML, JavaScript, CSS
To round the player in the css file simply just copy this
-webkit-border-top-right-radius: 5px; -moz-border-radius-topright: 5px; border-top-right-radius: 5px; -webkit-border-bottom-right-radius: 5px; -moz-border-radius-bottomright: 5px; border-bottom-right-radius: 5px; -webkit-border-bottom-left-radius: 5px; -moz-border-radius-bottomleft: 5px; border-bottom-left-radius: 5px; -webkit-border-top-left-radius: 5px; -moz-border-radius-topleft: 5px; border-top-left-radius: 5px;
and put it under (highlighted line)
#player { background-color:#4CBB17; width:400px; margin-left:300px; padding:5px; box-sizing:border-box; background:url(Textures/background.png); }
Sure thing in the #player section of the css go to the bg(background.png – Image), add the /* to filter out the lines of the unwanted code, then in the css on the #player constructor add this.
-webkit-box-shadow: 0px 0px 13px rgba(0,0,0,.3),inset 0 1px 0px rgba(255,255,255,.2); -moz-box-shadow: 0px 0px 13px rgba(0,0,0,.3),inset 0 1px 0px rgba(255,255,255,.2); box-shadow: 0px 0px 13px rgba(0,0,0,.3),inset 0 1px 0px rgba(255,255,255,.2); border: bold white; -ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=100)"; filter: alpha(opacity=100); opacity: 1; -webkit-box-shadow: inset 0 1px 0px rgba(255,255,255,.2); -moz-box-shadow: inset 0 1px 0px rgba(255,255,255,.2); box-shadow: inset 0 1px 0px rgba(255,255,255,.2); background: #f5f6f6; /* Old browsers */ background: -moz-linear-gradient(top, #f5f6f6 0%, #dbdce2 21%, #b8bac6 49%, #dddfe3 80%, #f5f6f6 100%); /* FF3.6-15 */ background: -webkit-linear-gradient(top, #f5f6f6 0%,#dbdce2 21%,#b8bac6 49%,#dddfe3 80%,#f5f6f6 100%); /* Chrome10-25,Safari5.1-6 */ background: linear-gradient(to bottom, #f5f6f6 0%,#dbdce2 21%,#b8bac6 49%,#dddfe3 80%,#f5f6f6 100%); /* W3C, IE10+, FF16+, Chrome26+, Opera12+, Safari7+ */ filter: progid:DXImageTransform.Microsoft.gradient( startColorstr='#f5f6f6', endColorstr='#f5f6f6',GradientType=0 ); /* IE6-9 */
be sure to add it under the code in
#player { width:400px; margin-left:300px; padding:5px; box-sizing:border-box; -webkit-border-top-right-radius: 15px; -moz-border-radius-topright: 15px; border-top-right-radius: 15px; -webkit-border-bottom-right-radius: 15px; -moz-border-radius-bottomright: 15px; border-bottom-right-radius: 15px; -webkit-border-bottom-left-radius: 15px; -moz-border-radius-bottomleft: 15px; border-bottom-left-radius: 15px; -webkit-border-top-left-radius: 15px; -moz-border-radius-topleft: 15px; border-top-left-radius: 15px; }
HTML tags used in the example I provided for creating an audio player in HTML:
<!DOCTYPE html>
: Defines the document type and version of HTML being used.<html>
: The root element of an HTML page.<head>
: Contains metadata about the document, such as the character encoding, title, and linked stylesheets.<meta charset="UTF-8">
: Specifies the character encoding for the document as UTF-8, which supports a wide range of characters and symbols.<meta name="viewport" content="width=device-width, initial-scale=1.0">
: Sets the viewport properties for responsive web design.<title>
: Defines the title of the web page, which appears in the browser’s title bar or tab.<link rel="stylesheet" href="styles.css">
: Links an external CSS stylesheet (styles.css) to apply styles to the HTML content.<body>
: Contains the visible content of the web page.<div class="audio-player">
: A<div>
element with the class “audio-player” used as a container for the audio player components.<audio id="audio" controls>
: The<audio>
element is used to embed audio content in the web page. Theid
attribute is set to “audio” for JavaScript interaction, and thecontrols
attribute adds standard audio controls (play, pause, volume) to the player.<source src="your-audio-file.mp3" type="audio/mpeg">
: Inside the<audio>
element, this<source>
element specifies the audio file to be played (“your-audio-file.mp3”) and its MIME type (audio/mpeg)."Your browser does not support the audio element."
: A message displayed if the browser does not support the<audio>
element.<div class="controls">
: A<div>
element with the class “controls” to contain the playback controls.<button id="play-pause">Play</button>
: A<button>
element with the id “play-pause” used to play and pause the audio. Initially displays “Play.”<input id="volume" type="range" min="0" max="1" step="0.01" value="1">
: An<input>
element with the id “volume” that’s a range input. It allows users to adjust the volume level with values ranging from 0 to 1.<script src="script.js"></script>
: Includes an external JavaScript file (script.js) to add interactivity to the audio player.
These HTML tags and elements work together to create a simple audio player with play/pause and volume controls. The CSS file (styles.css) provides styling, and the JavaScript file (script.js) adds functionality to control audio playback and volume adjustments.
Make sure to include your audio file in the same directory as your HTML file or provide the correct path to the audio file in the <source>
element.
Download source code of audio player in HTML, JavaScript, CSS
Download source code of Simple audio player in HTML, JavaScript, CSS: Simple audio player in HTML, JavaScript, CSS
Conclusion
In conclusion, the provided code demonstrates how to create a basic audio player using HTML, JavaScript, and CSS. Here’s a summary of the key components:
- HTML: The HTML file (index.html) defines the structure of the web page and includes the following elements:
<audio>
: Embeds the audio content and provides standard playback controls.<source>
: Specifies the audio file source and its MIME type.<button>
: A button for playing and pausing the audio.<input>
: A range input for adjusting the audio volume.
- CSS: The CSS file (styles.css) is responsible for styling the audio player and controls.
- JavaScript: The JavaScript file (script.js) adds interactivity to the audio player by handling events such as play/pause button clicks and volume adjustments.
Together, these components create a functional audio player that allows users to play, pause, and adjust the volume of an audio file. You can customize the code and styling to fit your specific design requirements and audio content.
Search Keywords
- Create simple audio player in HTML, JavaScript, CSS
- Audio player using HTML, JavaScript, CSS
Other Popular Projects 1. Create a Classic Tic-Tac-Toe Game in Python, Download Source Code 2. Create a Notepad using Python, Download Source Code 3. Create Fruit Ninja Game in Python, Download Source Code 4. Create School Management System with PHP & MySQL 5. Create Calculator Program in Python, Download Source Code